Building robust and reliable applications is more than just writing code—it’s about ensuring that the entire development and deployment lifecycle is efficient, repeatable, and error-free. In this post, we’ll share how we applied ACDC principles while developing and deploying an SPFx web part.
Core Tech Stack
- SPFx: Framework for creating client-side web parts in SharePoint.
- React: For building the user interface.
- TypeScript: For type-safe development.
- Azure DevOps: For CI/CD pipeline implementation.
Applying Development Best Practices
Version Control with Git
To ensure collaboration and proper tracking of changes, We used a Git workflow that included:
- Feature Branching: Each feature or bug fix was developed on its own branch.
- Test and Main Branches
- Pull Requests (PRs): Changes reviewed through PRs to ensure quality and encourage peer review.
- Main Branch Protections: Enforced policies such as requiring PR reviews and passing CI checks before merging to
main
.
Code Quality Checks
Code quality was enforced through:
- ESLint and Prettier: Automated linting and formatting checks.
- TypeScript: Leveraged TypeScript to catch type-related errors during development.
- React Project Structure:
assets/
: For static assets such as images or styles. This structured approach improves maintainability, readability, and scalability of the codebase.
components/
: For reusable UI components.
services/
: For logic interacting with APIs or SharePoint data.
utils/
: For utility functions.
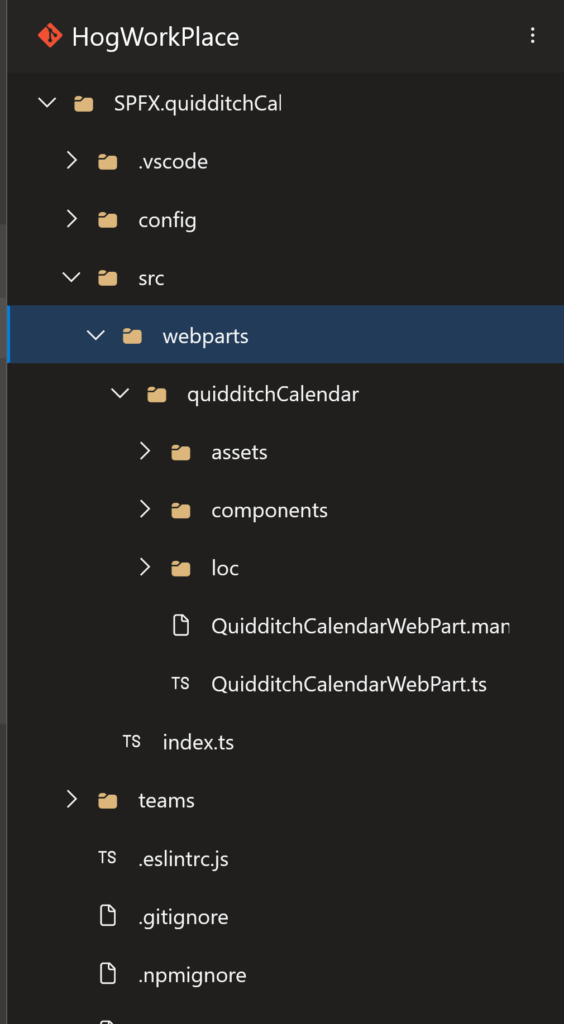
Overview of the Pipeline
CI/CD Pipeline: Automating Build and Release
The CI/CD pipeline was set up in Azure DevOps and included the following stages:
1. Build Stage
- Trigger: The pipeline runs automatically on every push to
main
. - Tasks:
- Install dependencies:
npm install
- Build the SPFx package:
gulp bundle --ship
- Package the solution:
gulp package-solution --ship
- Linting: Ensures no build-breaking errors.
- Copy files from a source folder to a target folder
- Publish build artifacts to Azure Pipelines
- Install dependencies:
Our YAML for the main branch:
# Node.js
# Build a general Node.js project with npm.
# Add steps that analyze code, save build artifacts, deploy, and more:
# https://docs.microsoft.com/azure/devops/pipelines/languages/javascript
trigger:
- master
pool:
vmImage: ubuntu-latest
steps:
- task: NodeTool@0
inputs:
versionSpec: '18.20.0'
displayName: 'Install Node.js'
- task: Npm@1
inputs:
command: 'install'
workingDir: 'SPFX.quidditchCal'
- task: gulp@0
displayName: 'gulp bundle'
inputs:
gulpFile: SPFX.quidditchCal/gulpfile.js
targets: bundle
arguments: '--ship'
- task: gulp@0
inputs:
gulpFile: 'SPFX.quidditchCal/gulpfile.js'
targets: 'package-solution'
arguments: '--ship'
gulpjs: 'node_modules/gulp/bin/gulp.js'
enableCodeCoverage: false
- task: CopyFiles@2
displayName: 'Copy Files to: drop'
inputs:
Contents: '**/solution/*.sppkg'
TargetFolder: '$(Build.ArtifactStagingDirectory)/drop'
OverWrite: true
flattenFolders: true
- task: PublishBuildArtifacts@1
displayName: 'Publish Artifact: ClientsideSolutions'
inputs:
PathtoPublish: '$(Build.ArtifactStagingDirectory)/drop'
ArtifactName: ClientsideSolutions
2. Release Stage
- Artifacts: The SPFx
.sppkg
file is published as a pipeline artifact. - Deployment Tasks:
- Automatically upload the
.sppkg
file to the SharePoint App Catalog using a PowerShell script.
- Automatically upload the
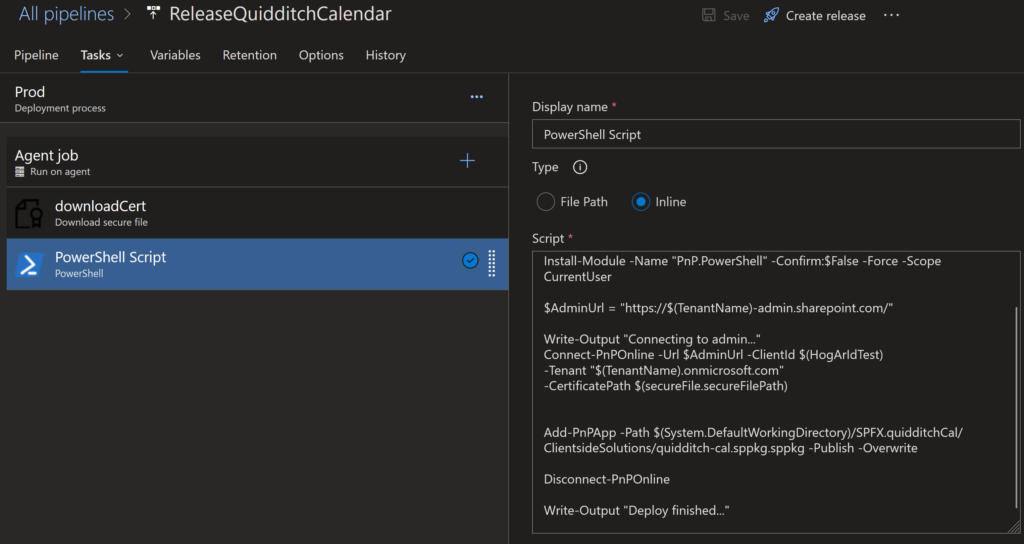
Different Stages depending on branch and environment:
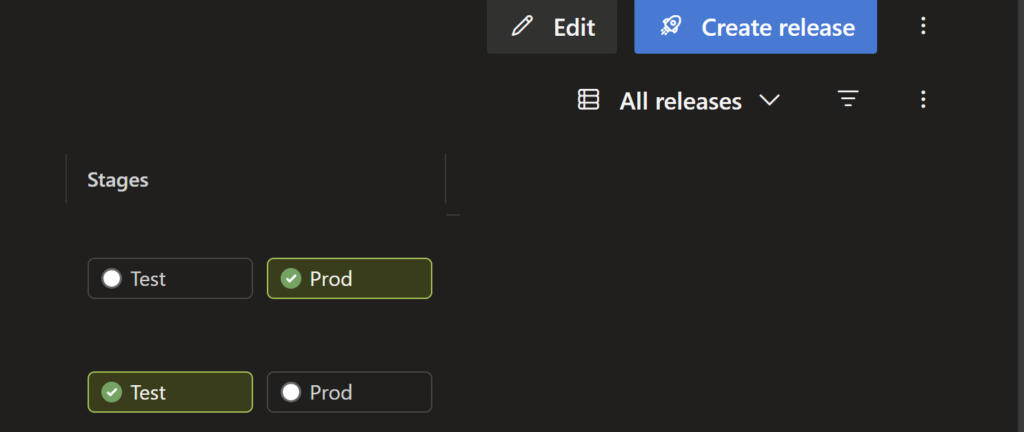
Key ACDC Principles in Action
Automation
- Every build and deployment step is automated, reducing the chances of human error.
- Triggers ensure that builds happen on every push to
main
, keeping the solution production-ready.
Continuous Testing
- Static Code Analysis:
- TypeScript for type checking.
- ESLint to check for linting issues: consistent code quality adhering to standards.
Continuous Delivery and Deployment
- Artifacts are built and ready for deployment in every run.
- Automatic deployment to the SharePoint App Catalog ensures faster delivery to end-users.